String manipulation is often necessary in UiPath Studio development.
In this article, I will show you how to join, get, add, replace, delete, convert, and split strings, with samples.
This is a very long article, so please touch (or click) the table of contents to jump to the chapter you want to know.
\Save during the sale period!/
Take a look at the UiPath course on the online learning service Udemy
*Free video available
Related Articles Learn the Creation Techniques f UiPath robotics creation with Udemy’s online courses that take it up a notch
This site was created by translating a blog created in Japanese into English using the DeepL translation.
Please forgive me if some of the English text is a little strange
- String binding
- Getting a string
- Get the length of a string (Length)
- Extract from the middle to the end of a string (Substring)
- Extract from the back of a string (Substring, Length)
- Extracting a part of a string (Substring)
- Search for a specified string from before and get its position (IndexOf)
- Search for the specified string from behind and get the position (LastIndexOf)
- Adding a String
- Add a string to the specified position (Insert)
- Add the specified character before the first character until the specified number of digits are reached (PadLeft).
- Adds the specified character after the last character until the specified number of digits are reached (PadRight).
- Add a new line (Environment.NewLine, vbCrLf )
- String substitution
- Deleting a string
- Delete a specified number of characters from the left of a string (Substring)
- Remove a specified number of characters from the right side of a string (Substring, Length)
- Removes the string from the specified positionRemove)
- Remove a specified number of characters from a specified position in a string (Remove)
- Remove white space before and after a string (Trim)
- Remove white space in front of a string (LTrim)
- Remove white space after a string (RTrim)
- Remove a specified string from a string (Replace)
- Remove newlines from a string (Replace)
- Convert string
- Splitting a string
- summary
String binding
String binding(+)
To join strings, put a + between characters.
Code to combine String type variables “str1” and “str2” with “-” and “xyz” strings
str1+str2+"-"+"xyz"
・Sample Process
・Execution result
Combine strings (Concat)
The concatenation of strings can also be done using the Concat function.
]Code to combine String type variables “str1” and “str2” with “-” and “xyz” strings
String.Concat(str1,str2,"-","xyz")
・Sample Process
・Execution result
Getting a string
Get the length of a string (Length)
The length of the string is obtained by Length.
Code to get the character length of the variable “str1” of type String.
str1.Length
・Sample Process
・Execution result
Extract from the middle to the end of a string (Substring)
If you want to extract a string from the middle to the end, use SubString.
Code to get the third character (position 2) of the variable “str1” of type String.
str1.String(2)
- First value in (): target position *Note that the first character position is 0.
・Sample Process
・Execution result
・Other samples and execution results
Left side of // is code, right side of // is output result
str1 = "abcxyz"
str1.Substring(0) // abcxyz
str1.Substring(1) // bcxyz
str1.Substring(2) // cxyz
str1.Substring(3) // xyz
str1.Substring(4) // yz
str1.Substring(5) // z
Extract from the back of a string (Substring, Length)
To extract from the back of a string, combine SubString and Length.
Code to get the two characters from the back of the variable “str1” of type String.
str1.Substring(str1.Length-2)
- First value in (): Subtract the number of characters to be retrieved from the number of characters in the target string (Length).
・Sample Process
・Execution result
・Other samples and execution results
Left side of // is code, right side of // is output result
str1 = "abcxyz"
str1.Substring(str1.Length-1) // z
str1.Substring(str1.Length-2) // yz
str1.Substring(str1.Length-3) // xyz
str1.Substring(str1.Length-4) // cxyz
str1.Substring(str1.Length-5) // bcxyz
Extracting a part of a string (Substring)
You can also use SubString to extract a part of a string.
Code to get three characters from the second character (position 1) of the variable “str1” of type String.
str1.String(1,3)
- First value in (): target position *Note that the first character position is 0.
- Second value in (): number of characters to retrieve
・Sample Process
・Execution result
・Other samples and execution results
Left side of // is code, right side of // is output result
str1 = "abcxyz"
str1.Substring(1,2) // bc
str1.Substring(2,3) // cxy
str1.Substring(2,4) // cxyz
str1.Substring(3,2) // xy
str1.Substring(4,2) // yz
Search for a specified string from before and get its position (IndexOf)
To retrieve the position of a specified string by searching from before, use IndexOf.
Code to get the first position of the string “bc” in the String type variable “str1”.
str1.IndexOf("bc")
- The first value in (): the string to search for
・Sample Process
・Execution result
・Other samples and execution results
Left side of // is code, right side of // is output result
str1 = "abcxyzabc"
str1.IndexOf("a") // 0
str1.IndexOf("b") // 1
str1.IndexOf("c") // 2
str1.IndexOf("bcx") // 1
str1.IndexOf("x") // 3
str1.IndexOf("y") // 4
str1.IndexOf("xy") // 3
str1.IndexOf("j") // -1
Search for the specified string from behind and get the position (LastIndexOf)
To retrieve the position of a specified string by searching from behind, use LastIndexOf.
Code to get the last position of the string “bc” in the variable “str1” of type String.
str1.LastIndexOf("bc")
- The first value in (): the string to search for
・Sample Process
・Execution result
・Other samples and execution results
Left side of // is code, right side of // is output result
str1 = "abcdeあいabcde"
str1.LastIndexOf("a") //6
str1.LastIndexOf("b") //7
str1.LastIndexOf("c") //8
str1.LastIndexOf("cxy") //2
str1.LastIndexOf("x") //3
str1.LastIndexOf("y") //4
str1.LastIndexOf("xy") //3
str1.LastIndexOf("j") //-1
Adding a String
Add a string to the specified position (Insert)
To add a string to the specified position, use Insert.
Code to add “@” after the third character of the String variable “str1”.
str1.Insert(3,"@")
- First value in (): position to add
- The second value in (): the string to be added
・Sample Process
・Execution result
・Other samples and execution results
Left side of // is code, right side of // is output result
str1 = "abcxyz"
str1.Insert(0,"@") // @abcxyz
str1.Insert(1,"@") // a@bcxyz
str1.Insert(2,"@") // ab@cxyz
str1.Insert(3,"@") // abc@xyz
str1.Insert(4,"@") // abcx@yz
str1.Insert(5,"@") // abcxy@z
str1.Insert(6,"@") // abcxyz@
Add the specified character before the first character until the specified number of digits are reached (PadLeft).
Use PadLeft to add the specified character before the first character until the specified number of digits is reached.
Code for adding leading zeros to a variable of type String “str1” until the number of digits reaches 10
str1.PadLeft(10,"0"c)
- First value in (): number of digits
- (Second value in parentheses: String to be added *Add c to the end of the string to convert it to Char type
・Sample Process
・Execution result
・Other samples and execution results
Left side of // is code, right side of // is output result
str1 = "1234"
str1.PadLeft(5,"0"c) // 01234
str1.PadLeft(6,"0"c) // 001234
str1.PadLeft(7,"0"c) // 0001234
str1.PadLeft(7,"@"c) // @@@1234
Adds the specified character after the last character until the specified number of digits are reached (PadRight).
Use PadRight to add the specified character before the first character until the specified number of digits are reached.
Code for adding backward 0’s to the String variable “str1” until the number of digits reaches 10.
str1.PadRight(10,"0"c)
- First value in (): number of digits
- (Second value in parentheses: String to be added *Add c to the end of the string to convert it to Char type
・Sample Process
・Execution result
・Other samples and execution results
Left side of // is code, right side of // is output result
str1 = "1234"
str1.PadRight(5,"0"c) // 12340
str1.PadRight(6,"0"c) // 123400
str1.PadRight(7,"0"c) // 1234000
str1.PadRight(7,"@"c) // 1234@@@
Add a new line (Environment.NewLine, vbCrLf )
NewLine or vbCrLf can be used to add a new line to a string in UiPath.
Code to add a new line between the strings ab and cd
"ab"+Environment.NewLine +"cd"
Or you can use the following code to get the same result.
"ab"+vbCrLf +"cd"
・Sample Process No1
*The equation for the first activity is
str1 = “ab”+Environment.NewLine +”cd”
・Execution result No1
・Sample Process No2
・Execution result No2
String substitution
Replace the specified string with another string (Replace)
To replace the specified string with another string, use Replace.
Code to replace “bc” in the String variable “str1” with “KLM” and output it
str1.Replace("bc","KLM")
- The first value in (): the string to be replaced
- The second value in (): the string to be replaced
・Sample Process
・Execution result
・Other samples and execution results
Left side of // is code, right side of // is output result
str1 = "abcxyzabcde"
str1.Replace("a","1") // 1bcxyz1bcde
str1.Replace("a","123") // 123bcxyz123bcde
str1.Replace("ab","1") // 1cxyz1cde
str1.Replace("bc","789") // a789xyza789de
str1.Replace("x","9") // abc9yzabcde
str1.Replace("xyz","5") // abc5abcde
str1.Replace("xyz","56789") // abc56789abcde
Deleting a string
Delete a specified number of characters from the left of a string (Substring)
To remove a specified number of characters from the left side of a string, use Substring.
Code for deleting two characters from the left side of the variable “str1” of type String
str1.Substring(2)
- First value in (): number of characters to be deleted
・Sample Process
・Execution result
・Other samples and execution results
Left side of // is code, right side of // is output result
str1 = "abcxyz"
str1.Substring(0) // abcxyz
str1.Substring(1) // bcxyz
str1.Substring(2) // cxyz
str1.Substring(3) // xyz
str1.Substring(4) // yz
str1.Substring(5) // z
Remove a specified number of characters from the right side of a string (Substring, Length)
To delete a specified number of characters from the right side of a string, use Substring and Length. Substring is used to delete characters from the beginning until one character before the character to be deleted is obtained.
Code to delete two characters from the right side of the variable “str1” of type String
str1.Substring(0,str1.Length-2)
- The first value in parentheses (): 0 * Fixed at 0, the position of the first character
- Second value in parentheses(): Subtract the number of characters to be deleted from the number of characters in the target string.
・Sample Process
・Execution result
・Other samples and execution results
Left side of // is code, right side of // is output result
str1 = "abcxyz"
str1.Substring(0,str1.Length-1) // abcxy
str1.Substring(0,str1.Length-2) // abcx
str1.Substring(0,str1.Length-3) // abc
str1.Substring(0,str1.Length-4) // ab
str1.Substring(0,str1.Length-5) // a
Removes the string from the specified positionRemove)
Use Remove to remove the string after the specified position.
Code to delete the third and subsequent characters (position 2 and subsequent) of the variable “str1” of type String.
str1.Remove(2)
- First value in (): specified position to be deleted * Note that the position of the first character is 0.
・Sample Process
・Execution result
・Other samples and execution results
Left side of // is code, right side of // is output result
str1 = "abcxyz"
str1.Remove(1) // a
str1.Remove(2) // ab
str1.Remove(3) // abc
str1.Remove(4) // abcx
str1.Remove(5) // abcxy
Remove a specified number of characters from a specified position in a string (Remove)
Remove is also used to remove a specified number of characters from a specified position in a string.
Code to delete two characters from the second character (position 1) of the variable “str1” of type String.
str1.Remove(1,2)
- First value in (): Start deletion position * Note that the first character position is 0.
- Second value in (): number of characters to delete
・Sample Process
・Execution result
・Other samples and execution results
Left side of // is code, right side of // is output result
str1 = "abcxyz"
str1.Remove(1,2) //axyz
str1.Remove(1,3) //ayz
str1.Remove(1,4) //az
str1.Remove(1,5) //a
str1.Remove(2,1) //abxyz
str1.Remove(2,2) //abyz
str1.Remove(2,3) //abz
str1.Remove(2,4) //ab
str1.Remove(3,1) //abcyz
Remove white space before and after a string (Trim)
Use Trim to remove white space before and after the string.
Code to remove white space before and after the variable “str1” of type String
str1.Trim
・Sample Process
・Execution result
*Blank spaces before and after have been removed.
・Other samples and execution results
Left side of // is code, right side of // is output result
str1 = " abcxyz "
str1.Trim //abcxyz
str1 = " ab cx yz "
str1.Trim //ab cx yz
Remove white space in front of a string (LTrim)
Use LTrim to remove white space before a string.
Code to remove the blank space before the variable “str1” of type String.
Ltrim(str1)
・Sample Process
・Execution result
*The previous blank space has been removed.
・Other samples and execution results
Left side of // is code, right side of // is output result
str1 = " abcxyz "
LTrim(str1) //abcxyz
str1 = " abcxyz "
LTrim(str1) //abcxyz
str1 = " ab cx yz "
LTrim(str1) //ab cx yz
Remove white space after a string (RTrim)
Use RTrim to remove white space after a string.
Code to remove the white space after the variable “str1” of type String.
Rtrim(str1)
・Sample Process
・Execution result
*Removed trailing whitespace
・Other samples and execution results
Left side of // is code, right side of // is output result
str1 = " abcxyz "
RTrim(str1) // abcxyz
str1 = " abcxyz "
RTrim(str1) // abcxyz
str1 = " ab cx yz "
RTrim(str1) // ab cx yz
Remove a specified string from a string (Replace)
To remove a specified string from a string, use Replace.
By replacing the specified string with “no string”, you can remove the string.
Code for deleting “bc” from a variable of type String “str1
str1.Replace("bc","")
- First value in (): string to be deleted * String to be replaced
- Second value in (): “” * String after replacement.”” means no string.
・Sample Process
・Execution result
・Other samples and execution results
Left side of // is code, right side of // is output result
str1 = "abcxyzabcde"
str1.Replace("a","") //bcxyzbcde
str1.Replace("b","") //acxyzacde
str1.Replace("c","") //abxyzabde
str1.Replace("bc","") //axyzade
str1.Replace("x","") //abcyzabcde
str1.Replace("y","") //abcxzabcde
str1.Replace("xy","") //abczabcde
str1.Replace("d","") //abcxyzabce
str1.Replace("e","") //abcxyzabcd
str1.Replace("cde","") //abxyzab
str1.Replace("k","") //abcxyzabcde
Remove newlines from a string (Replace)
Use Replace to remove newlines from a string.
The line breaks can be removed by replacing Chr(13) and Chr(10), which represent line breaks, with “no string”.
Code to remove line feeds from the String variable “str1”
str1.Replace(Chr(13), "").Replace(Chr(10), "")
・Sample Process
・Text file to read
・Execution result
Convert string
Convert a string to a number (Parse)
To convert a string to a number (Integer), use Parse.
Code to convert the variable “str1” of type String to a numeric value (Integer)
Integer.Parse(str1)
・Sample Process
・Execution result
Converting a number to a string (ToString)
To convert a number (Integer) to a string, use ToString.
Code to convert an Int variable “int1” to a string
int1.ToString
・Sample Process
* int1 and int2 are variables of type Int
・Execution result
Convert lower case letters to upper case (ToUpper)
Use ToUpper to convert lowercase letters to uppercase.
Code to convert lowercase to uppercase for the variable “str1” of type String.
str1.ToUpper
.Sample Process
・Execution result
Convert uppercase to lowercase (ToLower)
To convert uppercase to lowercase, use ToLower.
Code to convert uppercase to lowercase for the variable “str1” of type String.
str1.ToLower
.Sample Process
・Execution result
Splitting a string
Split a string with specified characters (Split)
To split a string with specified characters, use Split.
Code to get the first character of the delimiter “,” in the String variable “str1
split(str1,",")(0)
- First value in Split(): target string
- Second value in Split(): string to be separated
- Second value in (): Position of the separated characters
・サンプルワークフロー
・実行結果
・Other samples and execution results
Left side of // is code, right side of // is output result
str1 = "abc,123,de"
split(str1,",")(0) //abc
split(str1,",")(1) //123
split(str1,",")(2) //de
str1 = "abc/123/de"
split(str1,"/")(0) //abc
split(str1,"/")(1) //123
split(str1,"/")(2) //de
summary
- The function used this time to manipulate the string is
+、Concat、Length、Substring、IndexOf、LastIndexOf、Insert、PadLeft、PadRight、Replace、Remove、Trim、LTrim、RTrim、Parse、ToString、ToUpper、ToLower、Split - The most frequently used functions are +、Length、Substring、Replace、ToString、Split. If you can use this function alone, you will be able to develop much more efficiently, so please learn it.
\Save during the sale period!/
Take a look at the UiPath course on the online learning service Udemy
*Free video available
Related Articles Learn the Creation Techniques f UiPath robotics creation with Udemy’s online courses that take it up a notch
same category UiPath
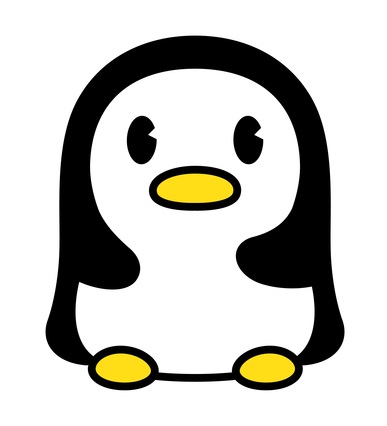
Japanese IT engineer with a wide range of experience in system development, cloud building, and service planning. In this blog, I will share my know-how on UiPath and certification. profile detail / twitter:@fpen17